Java Roadmap for Beginners: Basic to Advanced Complete Guide
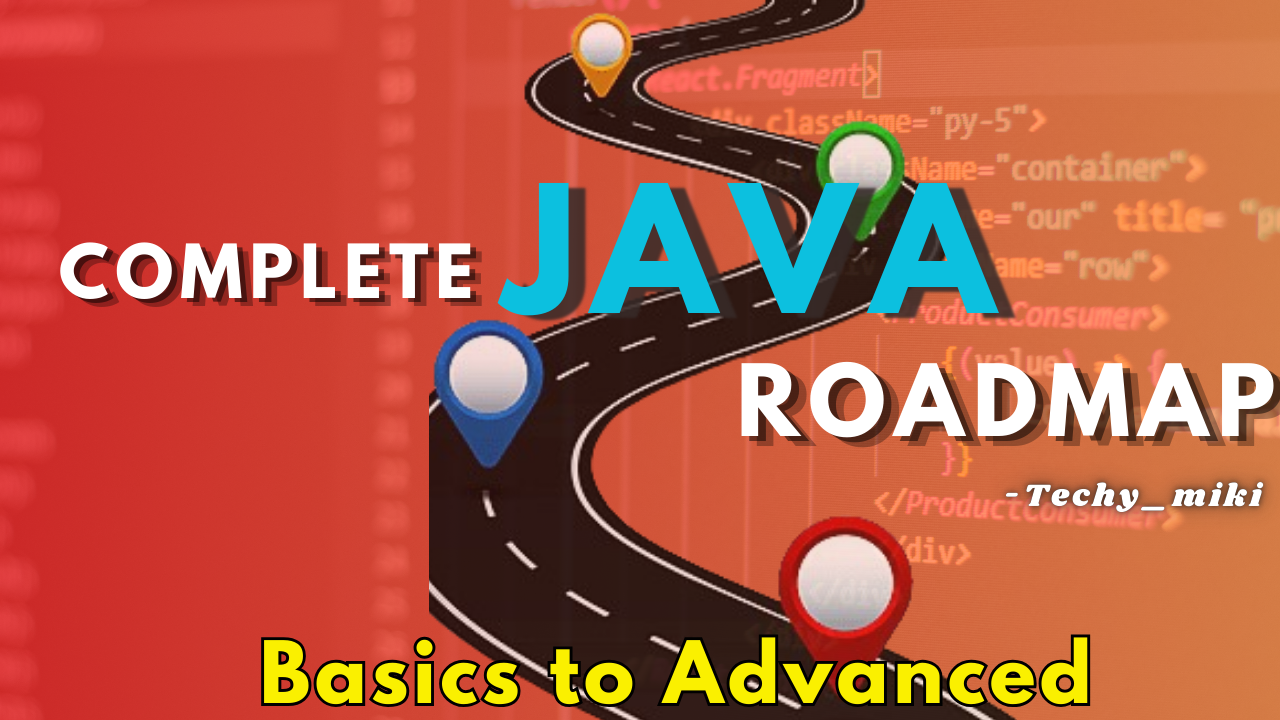
Why Learn Java?
Java has consistently been one of the most popular and widely-used programming languages. From large enterprise applications to Android development, Java is everywhere. It’s versatility, stability, and large community make it one of the best languages to learn if you are serious about programming. Let’s discuss the Complete Guide for Java here in this article.
In this roadmap, I’m going to breakdown everything you need to know to master java. Let us start from the Basic and explore all the steps required to complete our journey of learning Java. We will divide the roadmap into phases so that it can be easily understandable and easy to remember.
Let’s dive into our roadmap.
Phase 1: Java Fundamentals
1. Introduction to Java and Setup
- What is Java and it’s applications
- Install Java (JDK) and setup IDE
- Understanding JVM, JRE and JDK
2. Basic Syntax and Data Types
- Java syntax and structure, keywords
- Data types (int, float, char, boolean, double)
- Variables and operators (arithmetic, logical, relational)
- Input/output (print(), scanner class, etc.)
- Typecasting (implicit and explicit casting)
3. Control Flow Statements
- Conditional statements (if, else, else if, switch)
- Loops (for, while, do-while)
- Break, continue and return statements
- Nested loops and conditions
4. Functions/Methods
- Defining methods and calling methods
- Method overloading
- Return types and parameters
- static vs non-static methods
- Scope of variables (local, global)
Phase 2: Object Oriented Programming
5. Classes and Objects
- Class definition, creating objects
- Fields, methods, constructors
- this keyword, constructor overloading
- Access modifiers (public, private, protected, default)
6. Inheritance
- Types of Inheritance
- ‘super’ keyword
- Method overriding
- Constructor chaining in Inheritance
- ‘final’ keyword (methods and classes)
7. Polymorphism
- Compile-time polymorphism vs run-time polymorphism
- ‘instanceof’ keyword
- Upcasting and down casting
8. Abstraction and Interfaces
- Abstract classes and methods
- Interfaces, implementing multiple interfaces
- ‘default’ and ‘static’ methods in interfaces
9. Encapsulation
- Private data members, getters and setters
- Importance of Encapsulation in Java
Phase 3: Core Java
10. Java Collections Framework
- Lists (ArrayList, LinkedList), Sets (HashSet, TreeSet)
- Maps (HashMap, TreeMap)
- Queue and Dequeue
- Iterators, foreach loop
- Sorting collections using Comparator and Comparable
11. Exception Handling
- ‘try’, ‘catch’, ‘finally’ blocks
- Creating custom Exceptions
- Checked vs Unchecked exceptions
- ‘throw’ vs ‘throws’ keyword
12. Input / Output in Java
- Working with files (FileReader, FileWriter, BufferedReader, BufferedWriter)
- Reading and writing files using ‘File’ class
- Serialization and Deserialization
13. Multi-Threading
- Creating Threads (Thread class and Runnable interface)
- Thread lifecycle and states
- Synchronization, inter-thread communication (wait(), notify(), notifyAll())
- Deadlock and thread safety
Phase 4: Advanced Java
14. Java Generics
- Type parameters and wildcards
- Generic classes and methods
- Bounded type in generics
15. Lambda Expressions and Functional Programming
- Intro to Lambda Expressions
- Functional Interfaces (Consumer, Supplier, Function, Predicate)
- Method references and constructor references
16. Streams API
- Stream creation and pipeline
- Intermediate operations (filter(), map(), distinct())
- Terminal operations (forEach(), reduce(), collect())
Phase 5: Practical Projects and Frameworks
17. Build Real World Projects
- Simple CRUD application
- Library management system
- E-commerce product catalog system
- Banking Management System
18. Spring Framework
- Spring Boot basics and setup
- Dependency injection, inversion of control
- RESTful webservices with Spring Boot
- Connecting to database with Spring Data JPA
19. Hibernate Framework
- Introduction to ORM (Object-Relational Mapping)
- Hibernate basics (SessionFactory, Sessions)
- Mappings (one-to-one, one-to-many, many-to-one)
- Hibernate with Spring Boot
Phase 6: Data Structures and Algorithms in Java
20. Data Structures
- Array, Linked Lists, Stacks, Queues
- Trees (Binary Trees, Binary Search Trees, Heaps)
- Graphs (BFS, DFS)
21. Algorithms
- Sorting (Bubble Sort, Quick Sort, Merge Sort)
- Searching (Binary Search)
- Recursion and Dynamic Programming
- Greedy Algorithms
So guys, this is the complete step-by-step roadmap that will definitely help you to learn Java from very basic to advanced level.
Usually Time taken to learn java completely using this roadmap will be 6-8 months based on your allocation of time for learning. If you prioritize most of the time for this then you can learn more efficiently and easily within the stipulated time period or else it might cross the estimated time period and your learning journey may struck in between.
I recommend you prioritize your time for enhancing your skills so that it will be beneficial for you in the future.
If you like our content, please let us know in comments and if you have any queries reach out to us from contact page.
Thank you,
-Vinay neeradi.
I want SQL complete notes pdf
sure, we will upload very soon